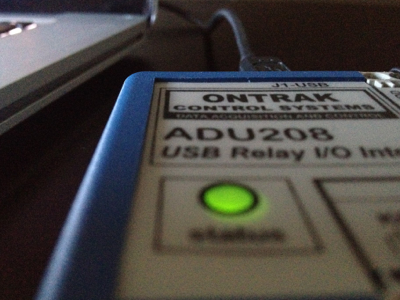
Building on the project in part 1, we’re going to
expand our project to extract the device’s Model and Serial Number!
Once again, I’ll be using an ONTRAK ADU208 as my test device, but
similar steps should work with other USB HID devices too.
In part 1, we created a function that gets called every time a matching
device is connected to the system. At present, this function simply logs
the device ID to the console. Let’s modify that function to store the
Name and Serial Number, then log them to the console:
|
|
// New USB device
specified in the matching dictionary has been added (callback function)
static
void Handle_DeviceMatchingCallback(void
*inContext,
IOReturn inResult,
void *inSender,
IOHIDDeviceRef inIOHIDDeviceRef){
// Retrieve the device name & serial number
NSString
*devName = [NSString stringWithUTF8String:
CFStringGetCStringPtr(
IOHIDDeviceGetProperty(inIOHIDDeviceRef,
CFSTR("Product")),
kCFStringEncodingMacRoman)];
NSString
*devSerialNumber = [NSString
stringWithUTF8String:
CFStringGetCStringPtr(
IOHIDDeviceGetProperty(inIOHIDDeviceRef,
CFSTR("SerialNumber")),
kCFStringEncodingMacRoman)];
// Log the device reference, Name, Serial
Number & device count
NSLog(@"\nONTRAK
device added: %p\nModel: %@\nSerial Number:%@\nONTRAK device count:
%ld",
inIOHIDDeviceRef,
devName,
devSerialNumber,
USBDeviceCount(inSender));
}
|
You’ll note that I’m using the IOHIDDeviceGetProperty
function to retrieve both the Model and the Serial
Number. IOHIDDeviceGetProperty returns a pointer to a
CFTypeRef, which is not necessarily a string. Since I’m
woking with an ONTRAK Control Systems ADU device, I knew
the type returned would be a string. In a production
application, it would be prudent to verify the type
returned before assigning it to a string.
At this point you can go ahead and run your project - it
will now log the device Reference, Model and Serial
Number when attached to your system!
Download the Xcode 4 project file
USBHIDpt2.zip.
This project was created on OS X 10.7.3 using Xcode
4.2.1.
Next time we will write to the device to open & close
its’ relays!
View the
next step.....Simple Read/Write For Ontrak
USB Data Acquisition Interfaces ( XCODE )
Back to Programming Page |