View the ADR series RS232 based Data
Acquisition products here.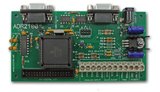
IMPORTANT:
For a tutorial on using the MSCOMM control
with older versions of Visual Basic See:
NOTE1 : This programming guide assumes the user has
a basic knowledge of Visual Basic programming. The teaching method used is to show a basic
example of a VB2010 program which communicates with an ADR board by sending and receiving
ASCII data, and then dissect the program to understand its operation.
NOTE2 : The procedure shown is
identical for VB2008, VB2010 and also runs on Express versions.
NOTE3 : The entire project can be
downloaded here.
ADR_SAMPLE_VB_dotNET.ZIP (98K)
ADR serial data acquisition interfaces
require the sending and
receiving of ASCII data via RS232 to operate. To communicate with the ADR boards using Visual Basic,
the SerialPort component must be utilized to allow serial data transfer via a serial port (
Com1-Com99). SerialPort is a component shipped with VB2008 and VB2010 and must be loaded
using the Toolbox/Components menu.
The following SerialPort VB2010 Example program is
used to communicate with an ADR112.
The ADR112 is an RS232 based data acquisition
interface that uses simple CR ( Chr(13) ) terminated ASCII strings to read two
12-bit analog input channels and control an 8-bit digital I/O port. The
program can be modified to work with any ONTRAK or other manufacturers
RS232 based product.
Figure
1: SerialPort VB2010 Example Form1
The program was built using a pull down menu for
COM port selection, an OPEN COM Port button, three
Send command buttons, and a text box to display received ASCII data. When run, the port is
selected using the Pull-Down Menu and enabled when the OPEN COM Port
button is clicked. The Send RDO button sends an ASCII " RDO" +
Chr(13) out on the COM port. The Chr(13) is required by the ADR112 to
tell it a command has been received. Two additional buttons send
SETPA0 and RESPA0 commands ( also terminated with Chr(13)) to set or
reset Bit PA0 on PORTA of the ADR112.
Figure 2 shows the program running with an
ADR112 connected to COM3. When the Send RD0 button was pressed,
the ADR112 returned ASCII 1711. This shows the ADR112 analog port
0 is at 1711/4095 X 5 =2.0891V. The number 4095 is the full scale
input of the ADR112 which is 5VDC.
Figure
2: SerialPort VB2010 Example Running
The entire program code is shown in Figure 3 and
Figure 4. The code is heavily commented and a few explanations are
provided below each figure.
Figure 3: Opening the COM Port and Some Housekeeping.
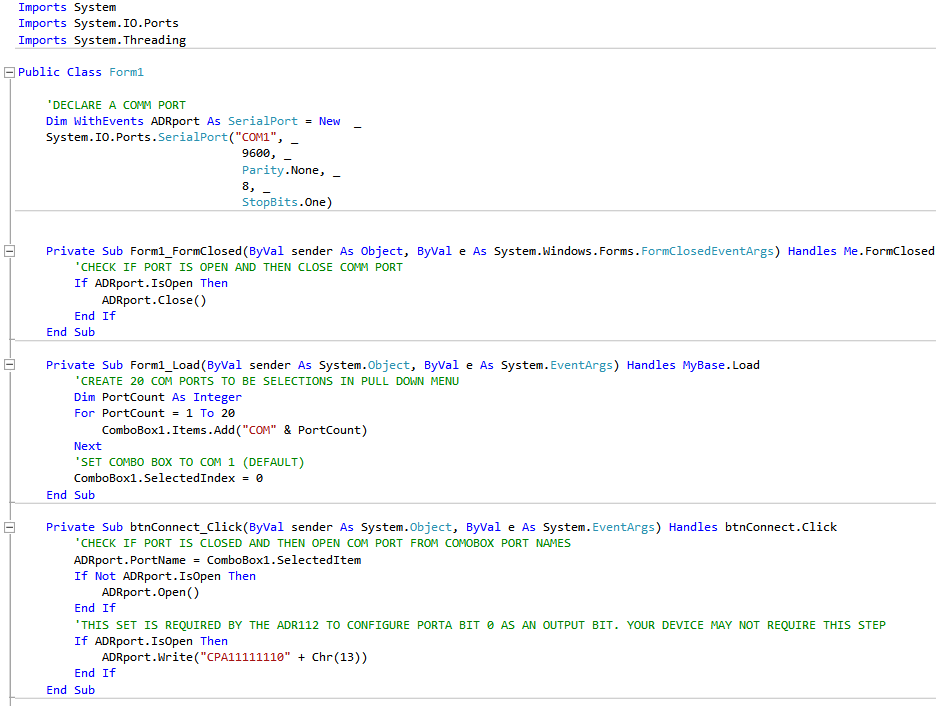
In Figure 3, we first declare a COM port assign it a label of ADRport.
Next, we check to see if the port is already open and if so we close
it.
The next block of code creates our pull down combobox with an array
of com ports from COM1 to COM20. This value can be increased if desired.
The last block of code is for the "OPEN COM Port" button. When the
button is pressed, the value in the combobox is used to open the
selected COM port and give it a label of ADRport. Further, the text
string "CPA11111110" + Chr(13) is sent out on the COM port to configure
the Digital I/O port so that PA0 is an output. This is required by the
ADR112 and depending on your hardware, initialization may or may not be
required.
Figure 4: Sending Commands and Receiving Data
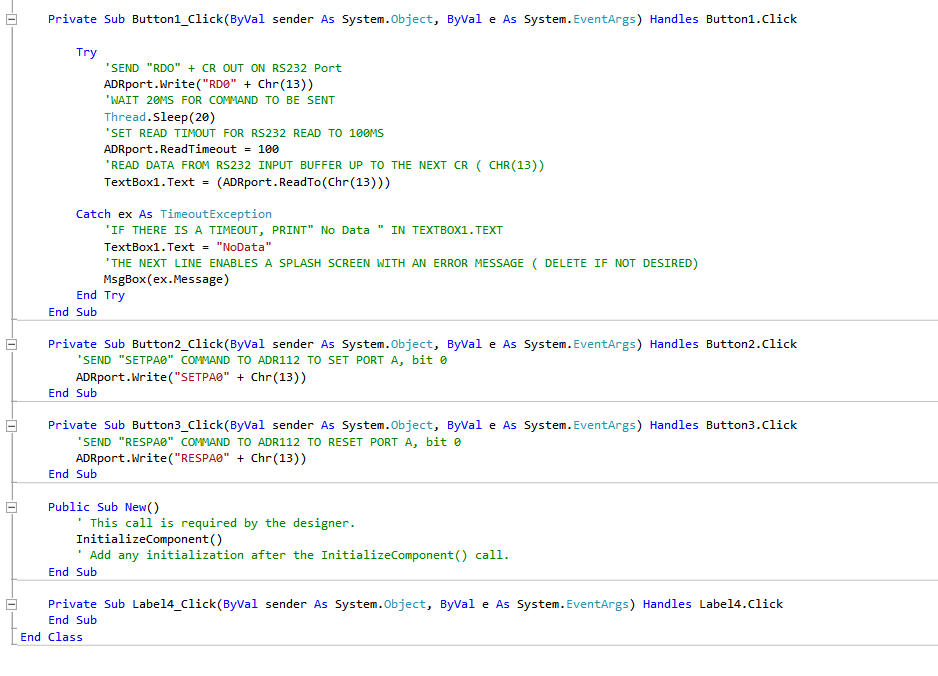
Figure 4 is where the sending and receiving of data happens under
control of the buttons on Form1.
The first block of code uses TRY CATCH to send an "RDO" + Chr(13) to
the ADR112 to retrieve the value of analog port AN0. the received data
is then placed in the text box on Form1.
Thread.Sleep(20) is used to force
the thread to wait 20ms. This gives time for the command to be sent out
on COM3 to the ADR112 and, for the response string to be received.
Catch ex As TimeoutException is used
to detect if there has been a timeout in the read of the COM port. If
there is a timeout, we print "No Data" in the text box and enable a
flash screen to show a timeout has occurred. We have set the
timeout to 100ms in our example. If you are running the program without
an ADR112 connected these events will be triggered.
The following two subroutines simply send either "SETPA0" +Chr(13) or
"RESPA0"+Chr(13) to set or reset PA0 on the
ADR112 depending on what button has been pressed.
FURTHER NOTES and Programming Hints;
1.USING VARIABLES - In many cases it may be desired
to send a string incorporating a command and some variable. For example, the
"MAddd" command outputs to port A, the integer value ddd. If ddd is a variable
named PV, a string to set the port to the value of this variable would look like;
ADRport.Write(" MA " & Str(PV) + Chr(13))
The "Str" function converts the variable to an ASCII
string and it is appended to "MA". The Chr(13) is a carriage return.
Good luck in your programming efforts.
The Ontrak Programming Team
2. CUT and PASTE - Here is
the entire program in a cut/paste format;
Imports System
Imports System.IO.Ports
Imports System.Threading
Public Class Form1
'DECLARE A COMM PORT
Dim WithEvents ADRport As SerialPort = New _
System.IO.Ports.SerialPort("COM1", _
9600, _
Parity.None, _
8, _
StopBits.One)
Private Sub Form1_FormClosed(ByVal sender As Object, ByVal e As
System.Windows.Forms.FormClosedEventArgs) Handles Me.FormClosed
'CHECK IF PORT IS OPEN AND THEN CLOSE COMM PORT
If ADRport.IsOpen Then
ADRport.Close()
End If
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles MyBase.Load
'CREATE 20 COM PORTS TO BE SELECTIONS IN PULL DOWN MENU
Dim PortCount As Integer
For PortCount = 1 To 20
ComboBox1.Items.Add("COM" & PortCount)
Next
'SET COMBO BOX TO COM 1 (DEFAULT)
ComboBox1.SelectedIndex = 0
End Sub
Private Sub btnConnect_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnConnect.Click
'CHECK IF PORT IS CLOSED AND THEN OPEN COM PORT FROM COMOBOX PORT NAMES
ADRport.PortName = ComboBox1.SelectedItem
If Not ADRport.IsOpen Then
ADRport.Open()
End If
'THIS SET IS REQUIRED BY THE ADR112 TO CONFIGURE PORTA BIT 0 AS AN
OUTPUT BIT. YOUR DEVICE MAY NOT REQUIRE THIS STEP
If ADRport.IsOpen Then
ADRport.Write("CPA11111110" + Chr(13))
End If
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles Button1.Click
Try
'SEND "RDO" + CR OUT ON RS232 Port
ADRport.Write("RD0" + Chr(13))
'WAIT 20MS FOR COMMAND TO BE SENT
Thread.Sleep(20)
'SET READ TIMOUT FOR RS232 READ TO 100MS
ADRport.ReadTimeout = 100
'READ DATA FROM RS232 INPUT BUFFER UP TO THE NEXT CR ( CHR(13))
TextBox1.Text = (ADRport.ReadTo(Chr(13)))
Catch ex As TimeoutException
'IF THERE IS A TIMEOUT, PRINT" No Data " IN TEXTBOX1.TEXT
TextBox1.Text = "NoData"
'THE NEXT LINE ENABLES A SPLASH SCREEN WITH AN ERROR MESSAGE ( DELETE IF
NOT DESIRED)
MsgBox(ex.Message)
End Try
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles Button2.Click
'SEND "SETPA0" COMMAND TO ADR112 TO SET PORT A, bit 0
ADRport.Write("SETPA0" + Chr(13))
End Sub
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles Button3.Click
'SEND "RESPA0" COMMAND TO ADR112 TO RESET PORT A, bit 0
ADRport.Write("RESPA0" + Chr(13))
End Sub
Public Sub New()
' This call is required by the designer.
InitializeComponent()
' Add any initialization after the InitializeComponent() call.
End Sub
Private Sub Label4_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles Label4.Click
End Sub
End Class
|